Play Google chrome dino game with python and pyautogui!
- 09-06-2018
- python
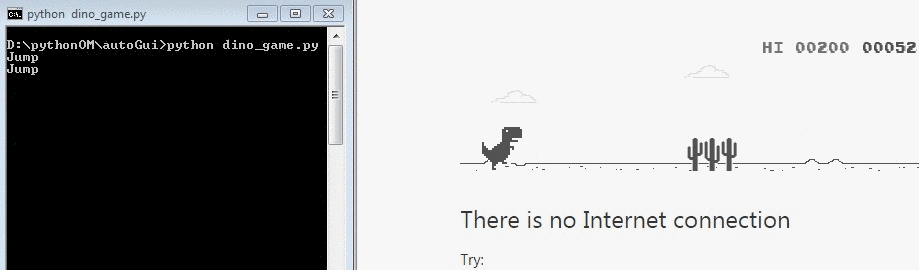
Share:
https://techconductor.com/blogs/python/play_google_dino_game_with_python.php
Copy
This is a great example of how we can easily use python pyautogui module to do some cool stuffs. Google chrome dino game is really popular and became a trademark of you are offline.
Here we will use python and pyautogui module to play the google chrome dino game. The process used here can also be modified to play other similar games.
Requirements:
- Python3
- pyautogui module
- pillow module
Getting ready:
- If you don't have python3 installed follow steps here.
- Install pyautogui using
pip install pyautogui
in a command prompt - Install pillow using
pip install pillow
in a command prompt.
How it works ?
- Takes screenshot of specified area.
- Checks for particular pixel colour of obstacles.
- If obstacles appears use pyautogui to virtually press space key.
- Repeat this again and again.
Step 1:
Go offline and open chrome. Grab it and stick it on one side of the window either left or right. So it won't move and changes its coordinates on the screen.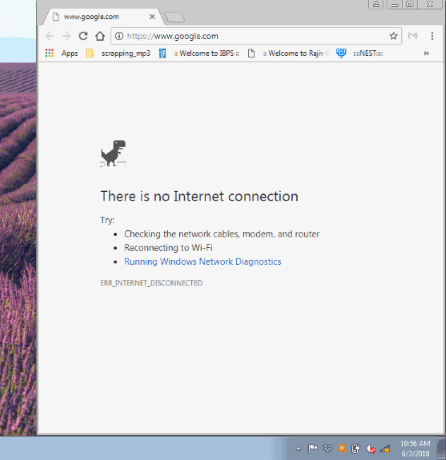
Step 2:
Take a screenshot when the plant is just a few centimetres away from the dino use PrtSc key. Then open Paint.net or any other image editing application and paste it Ctrl+V.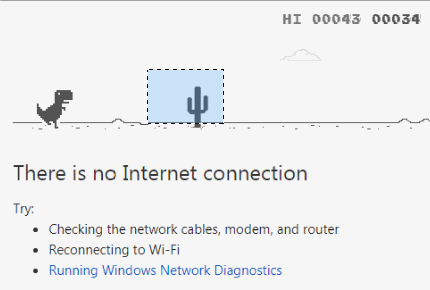
Step 3:
We need to mark a box where we want to detect the obstacles. Use the editor scale tool to mark the area. Then take a note of the two top-left and bottom-right corner points of the box area as indicated on the image below. These two coordinates will be used to make the box area in which we will detect obstacles. Then crop it.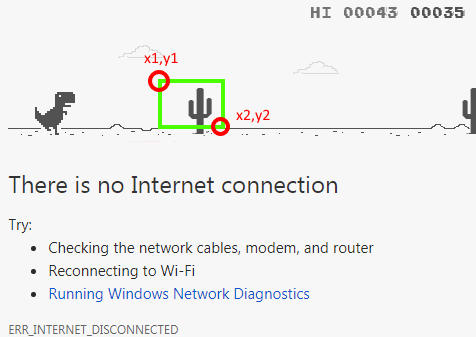
Step 4:
Zoom in as much as you can and take a note of two or three pixels coordinates on the plant as shown with Red dots on the image below.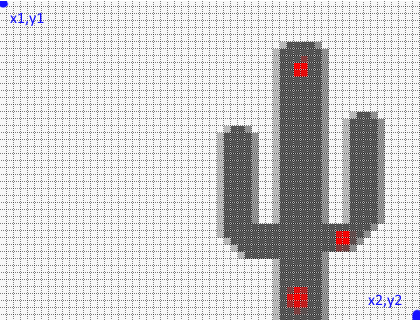
Stem 5:
Write the python code and save it asdino_game.py
import pyautogui import time import PIL.ImageGrab as ImageGrab pyautogui.FAILSAFE = True box_area1 = (919,229, 954, 270) #box coordinates(x1,y1, x2,y2) to detect obstacles pixel_plant1 = (21, 33) #pixel coordinates(x1,y1) of big plants pixel_plant2 = (19, 29) #pixel coordinates(x1,y1) of small plants pixel_plant3 = (17, 27) #pixel coordinates(x1,y1) of 3 in row plants def jump(): pyautogui.keyDown('space') time.sleep(0.05) pyautogui.keyUp('space') print('Jump') play = True while play: try: box = ImageGrab.grab(box_area1) p1 = box.getpixel(pixel_plant1) p2 = box.getpixel(pixel_plant2) p3 = box.getpixel(pixel_plant3) if p1 == (83, 83, 83) or p2 == (83, 83, 83) or p3 == (83, 83, 83): jump() time.sleep(0.06) except Exception as e: play = False print(e)
Step 6:
Box coordinates goes to the box_area1 variable. and plant coordinates goes to the pixel_plant variables.jump
function uses pyautogui.keyDown('space')
to virtually press down the Space key. pyautogui.keyUp('space')
to relese the Space key. And finally print Jump so we know its our code who made the dino jump.Step 7:
We make our mainwhile
loop and put all our game code inside it.box = ImageGrab.grab(box_area1)
takes a screenshot of the box we defined. p1 = box.getpixel(pixel_plant1)
gives the value of the pixel same goes for p2 and p3.With our
if
statement we check for the pixel value (83, 83, 83) because this is the value of the plant pixel colour.Whenever any of the three p1,p2 and p3 has the value (83, 83, 83) we call our
jump()
function.
Step 78
Run our code by browsing to the folder which contains our code file and open a commant prompt there windows user can use the shortcut Shift + Left Click and select open a command prompt here. Then usepython dino_game.py
to run it.
Result: small sample
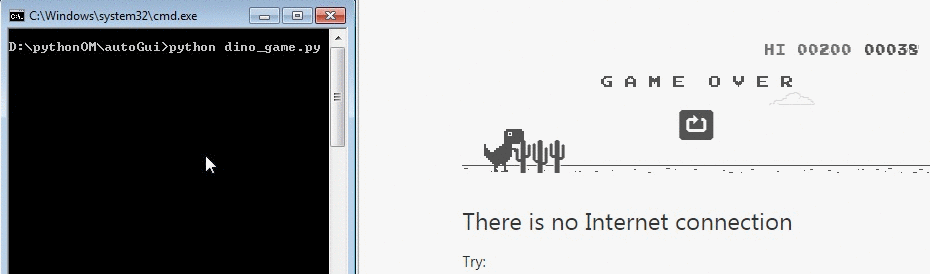
One test resulted a score over 600 using the same code.