Making an app/script with python to check Live Weather conditions!
- 26-06-2018
- python

Share:
Copy
The core of all live and forcast Weather checking apps and websites are weather API's, which provides all the weather information. There are many weather API's available online among them Yahoo Weather api is the most simplest and easy to start.
Yahoo weather api uses YQL (Yahoo Query Language) which is similar to SQL. We won't go deep into YQL as we only need one simple query to get all weather information. Nice thing about Yahoo is that we don't need any API key to access weather information as it's the most common case among all other API's out there.
To get the live weather information from Yahoo Weather API we need to make a GET request at query.yahooapis.com
along with the query written in YQL. The entire url is shown below:
https://query.yahooapis.com/v1/public/yql?q=select units,astronomy,atmosphere,wind, location,item from weather.forecast where woeid in (select woeid from geo.places(1) where text= london ) and u="c"&format=json
The whole string after ?q=
is query written in YQL. In the url text= london
holds the city name. The above url will return the json
response shown below:
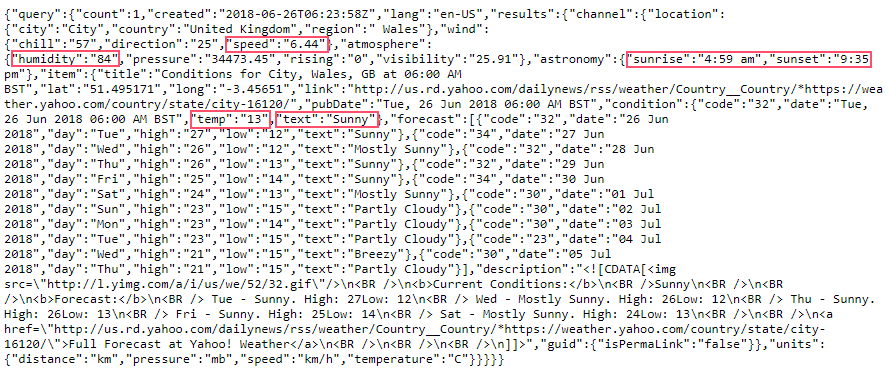
The json
response has all the information about the live weather conditions of the specified city. In this way we can easily get weather information about any city just by changing the city name in the url above.
The above method can be used in many ways. Here we will simply write a python script to check live weather information about a city and extract specific information from the json
response.
We will use python request
module to make a GET request to the Yahoo API with specified city name and then use python json
module to parse the data and extract specific information from the response.
Python code:
Githubfrom urllib.request import urlopen #import urlopen from request module import json #import json module print('-'*50) print('Please Enter your city:\n') city = str(input()) #asks for city name #url to request for weather imformation from yahoo weather api. City name is concatenated in the url url = 'https://query.yahooapis.com/v1/public/yql?q=select%20units,astronomy,atmosphere,wind,location,item%20from%20weather.forecast%20where%20woeid%20in%20(select%20woeid%20from%20geo.places(1)%20where%20text=%22'+city+'%22)%20and%20u=%22c%22&format=json' try: u = urlopen(url) #makes the GET request with the provided url page = u.read() #reads and stores the response to the page variable u.close() #connection is closed json_data = json.loads(page) #the response is only json data and no html so json.loads() method will load the json data into json_data variable. print('-'*50) #uncomment below line to see raw json data #print(json_data) #uncomment below line to see well structured or beautified json data in the command line #print(json.dumps(json_data, sort_keys=True, indent=2)) r_date = json_data['query']['results']['channel']['item']['condition']['date'] #extract date from json data temp = json_data['query']['results']['channel']['item']['condition']['temp'] #extract temperature from json data r_city = json_data['query']['results']['channel']['location']['city'] sunrise = json_data['query']['results']['channel']['astronomy']['sunrise'] sunset = json_data['query']['results']['channel']['astronomy']['sunset'] humidity = json_data['query']['results']['channel']['atmosphere']['humidity'] oveview = json_data['query']['results']['channel']['item']['condition']['text'] temp = json_data['query']['results']['channel']['item']['condition']['temp'] #print all the fetched information print('Report Date :::::: '+str(r_date)) print('City ::::::::::::: '+str(r_city)) print('Temperature :::::: '+str(temp)+' *C') print('Condition :::::::: '+str(oveview)) print('Sunrise :::::::::: '+str(sunrise)) print('Sunset ::::::::::: '+str(sunset)) print('Humidity ::::::::: '+str(humidity)+' %') print('-'*50) except Exception as e: print('Error:'+str(e))
The code above is nicely explained with comments on each line.
Result:
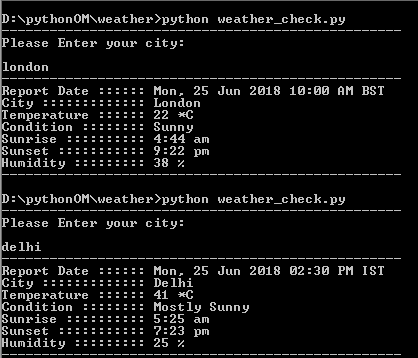
This is a general method and can be used in many ways. Later on we will see how I build a Web App located at techconductor.com/ weather using mostly AJAX, PHP and plain HTML & CSS.